Heres what the original df looks like: In the df object, the values are now sorted in ascending order based on the city08 column. When the index is a MultiIndex the Data analysis is commonly done with Pandas, SQL, and spreadsheets. Ranking the 2023 AFC East running backs: Which revamped backfield is Pandas rank () Method: Equivalent to ROW_NUMBER (), RANK (), DENSE_RANK Heres how to use na_postion in .sort_values(): Now any missing data from the columns you used to sort on will be shown at the top of your DataFrame. How would I count the number of '1's under label for each Paragraph (under paragraphA) and also the number of times these '1's appear in the top x results? Similar to how you were able to pass in a list of columns to sort by multiple columns, youre also able to pass in a list of boolean values to modify the sort order of the various columns. before sorting. By using our site, you What would happen if you used the following code: df.sort_values(by=[region, gender], ascending = [True, True, False]). Next, you'll see how to sort that DataFrame using 4 different examples. You can unsubscribe anytime. '1 ----'). 1 Answer Sorted by: 62 Here's one way to do it in Pandas-way You could groupby on Auction_ID and take rank () on Bid_Price with ascending=False So the end result would be: 1) Paragraph 1 | 3 (total 1s) | 2 (number of 1s in the first 3 rows for that paragraph) and so on, With my formula, what you obtain is: Paragraph 1 | 3 (total 1s) | 0.66 (percentage of 1s in the first 3 rows, in other words 2/3). Sorting and Ranking of Pandas Data Structures.. Site design / logo 2023 Stack Exchange Inc; user contributions licensed under CC BY-SA. See also numpy.sort() for more make model Alfa Romeo Spider Veloce 2000 19 4 Manual 5-spd 1985, Ferrari Testarossa 9 12 Manual 5-spd 1985, Dodge Charger 23 4 Manual 5-spd 1985, B150/B250 Wagon 2WD 10 8 Automatic 3-spd 1985, Subaru Legacy AWD Turbo 17 4 Manual 5-spd 1993. If youre familiar with Pythons built-in functions sort() and sorted(), then the inplace parameter available in the pandas sort methods might feel very similar. Missing values can often cause unexpected results. For this tutorial, youll need only a subset of the available columns. This allows you to establish a sorting hierarchy, where data are first sorted by the values in one column, and then establish a sort order within that order. We take your privacy seriously. The row index can be thought of as the row numbers, which start from zero. use ascending=False. It includes data structures such as Dataframes and Series for handling structured data. You can think of the index as the row numbers. The default value for this is True. To illustrate the use of na_position, first youll need to create some missing data. Syntax: DataFrame.rank (axis=0, method='average', numeric_only=None, na_option='keep', ascending=True, pct=False) Parameters: axis: 0 or 'index' for rows and 1 or 'columns' for Column. The following functions are used for this: Firstly, we need to ensure that the rows in both DataFrame follow the same order. By default, .sort_values() sorts your data in ascending order. You now know how to use two core methods of the pandas library: .sort_values() and .sort_index(). Pandas Value_counts to Count Unique Values. How to sort a Pandas DataFrame by multiple columns in Python? The parameter accepts a boolean value, meaning either True or False. .sort_index() also accepts na_position. To subscribe to this RSS feed, copy and paste this URL into your RSS reader. Hosted by OVHcloud. For Let's get started. the IDs with the highest value across groups would get ranks closer to 1). Now your DataFrame is sorted in descending order by the average MPG measured in city conditions. How to combine uparrow and sim in Plain TeX? if axis is 0 or index then by may contain index Series.sort_values: All missing values are sorted to the end of the row by default: With a DataFrame you can sort on both axes. With by you specify which column or row is to be sorted: You can also sort rows with axis=1 and by: DataFrame.rank and Series.rank assign ranks from one to the number of valid data points in an array: If ties occur in the ranking, the middle rank is usually assigned in each group. In this case, that would be the region. By default, missing values are sorted at the end of the sort values. and returning a float. Returns DataFrame or None DataFrame with sorted values or None if inplace=True. Learning pandas sort methods is a great way to start with or practice doing basic data analysis using Python. Sometimes you need to sort the dataset by indexes or columns. Interaction terms of one variable with many variables. When youre sorting multiple records that have the same key, a stable sorting algorithm will maintain the original order of those records after sorting. We then sorted the data by the 'sales' column in increasing order. Most commonly, data analysis is done with spreadsheets, SQL, or pandas. Why do Airbus A220s manufactured in Mobile, AL have Canadian test registrations? This is because quicksort is not a stable sorting algorithm, but mergesort is. 4. Lets sort by the values of the b columns. The code in this tutorial was executed using pandas 1.2.0 and Python 3.9.1. Contribute your expertise and make a difference in the GeeksforGeeks portal. You use .sort_values() to sort values in a DataFrame along either axis (columns or rows). See also numpy.sort() for more This article will discuss how to sort Pandas Data Frame using various methods in Python. For example, the EPAs emissions dataset also uses id to represent vehicle record IDs. levels and/or index labels. Find centralized, trusted content and collaborate around the technologies you use most. Specify list for multiple sort From an analysis standpoint, the MPG in city conditions is an important factor that could determine a cars desirability. Both rows and columns have indices, which are numerical representations of where the data is in your DataFrame. To change that behavior and have the missing data first in your DataFrame, set na_position to first. Similarly, your list of columns will be sorted from left to right in sort_values. Here are the commands to read the relevant columns of the fuel economy dataset into a DataFrame and to display the first five rows: By calling .read_csv() with the dataset URL, youre able to load the data into a DataFrame. Behavior of narrow straits between oceans. .sort_values() accepts a parameter named na_position, which helps to organize missing data in the column youre sorting on. By default, this parameter is set to last, which places NaN values at the end of the sorted result. What determines the edge/boundary of a star system? In this example, you sort the DataFrame by the city08 column, which represents city MPG for fuel-only cars: This sorts your DataFrame using the column values from city08, showing the vehicles with the lowest MPG first. This is helpful because it groups the cars in a categorical order and shows the highest MPG cars first. Using sorting, how would you get the second-highest sales across all regions? The pandas official documentation for sort_values is here and for .rank is here. Watch it together with the written tutorial to deepen your understanding: Sorting Data in Python With pandas. Find centralized, trusted content and collaborate around the technologies you use most. In this example, you sort your DataFrame by the make, model, and city08 columns, with the first two columns sorted in ascending order and city08 sorted in descending order. To show sorting for data frame, Im going to create a data named df. There are around 500 paragraphs and each is listed as a pair in the following format (sorted by paragraphA and ranked by highest prediction): I already sorted and grouped this (beforehand the paragraphs and predictions were in random order): This is a ranking problem where I tried to predict the likelihood that there was a link between paragraphs. Pandas: How to Calculate Rank in a GroupBy Object - Statology Heres what your DataFrame looks like when you sort on the column with missing data: To change that behavior and have the missing data appear first in your DataFrame, you can set na_position to first. If you want to change the logical sort order from the previous example, then you can change the order of the column names in the list you pass to the by parameter: Your DataFrame is now sorted by the model column in ascending order, then sorted by make if there are two or more of the same model. To find out more about using .map(), you can read Pandas Project: Make a Gradebook With Python & Pandas. However, its good to know that if your DataFrame does have NaN in either the row index or a column name, then you can quickly identify this using .sort_index() and na_position. The dataset contains eighty-three columns in total. Pandas sort_values () can sort the data frame in Ascending or Descending order. To get your new DataFrame back to the original order, you can use .sort_index(): Now the index is in ascending order. This can be helpful for visual inspection of the DataFrame. Try to solve them on your own first and then check your understanding by viewing the solution. See also DataFrame.sort_index Sort a DataFrame by the index. Connect and share knowledge within a single location that is structured and easy to search. Example 1: Sorting the Data frame in Ascending order, Example 2: Sorting the Data frame in Descending order, Example 3: Sorting Pandas Data frame by putting missing values first, Example 4: Sorting Data frames by multiple columns, Example 5: Sorting Data frames by multiple columns but different order, Python | Pandas DataFrame.fillna() to replace Null values in dataframe, Difference Between Spark DataFrame and Pandas DataFrame, Pandas Dataframe.to_numpy() - Convert dataframe to Numpy array, Convert given Pandas series into a dataframe with its index as another column on the dataframe. Lets sort the dataset according to the values of the year column. In this tutorial, you'll learn how to use the rank function including how to rank an entire dataframe or just a number of different columns. Sorting on the index has no impact on the data itself as the values are unchanged. Learning pandas sort methods is a great way to start with or practice doing basic data analysis using Python. This is because the default argument is 'na_position='last'. By clicking Accept all cookies, you agree Stack Exchange can store cookies on your device and disclose information in accordance with our Cookie Policy. Lecture 9. Next, you can sort the customers by descending sales, using sort_values. The parameter min, on the other hand, assigns the smallest rank in the group: default: assign the average rank to each entry in the same group, uses the minimum rank for the whole group, uses the maximum rank for the whole group, assigns the ranks in the order in which the values appear in the data, like method='min' but the ranks always increase by 1 between groups and not according to the number of same items in a group, Toggle navigation of Read, persist and provide data, Toggle navigation of Serialisation formats, Toggle navigation of Application Programming Interface (API), Toggle navigation of Data cleansing and validation, Toggle navigation of Manage code with Git, Toggle navigation of Manage data with DVC, Toggle navigation of Reproduce environments, Toggle navigation of Check and improve code quality and complexity, Create, update and delete files and directories, Array-oriented programming vectorisation, Introduction to the data structures of pandas, Converting Python data structures into pandas, Intake-GUI: Exploring data in a graphical user interface, Requests installation and sample application, Optimising PostgreSQL for GIS database objects, Data validation with Voluptuous (schema definitions), Assigning satellite data to geo-locations, Introduction to multithreading, multiprocessing and async, Use case 1: managing combinatorial installations, Use case 2: Python and other interpreted languages, Creating programme libraries and packages, Check and improve code quality and complexity. How to Convert Wide Dataframe to Tidy Dataframe with Pandas stack()? Your email address will not be published. In the examples above, we saw that the sort order defaulted to sort data in ascending order. python - Ranking order per group in Pandas - Stack Overflow Your original DataFrame has been modified, and the changes will persist. Returns DataFrame or None The original DataFrame sorted by the labels or None if inplace=True. For the next example, youll sort your DataFrame by its index in descending order. Spencer is data engineer who loves Python and automation. Please note: this is only applicable to DataFrames/Series with a monotonically increasing/decreasing index. Pandas Sorting Methods. Pandas - Group by and rank within group based on multiple columns How to Get Top 10 Highest or Lowest Values in Pandas - DataScientYst This means that we can sort one column in, say, ascending order and another in descending order. You could combine .sort_values() with .iloc: In this tutorial, you learned how to sort your Pandas DataFrame using the .sort_values() method. import pandas as pd This question does not meet Stack Overflow guidelines. This allows you to preserve the state of the data from when you read it from your file. If True, the resulting axis will be labeled 0, 1, , n - 1. By clicking Post Your Answer, you agree to our terms of service and acknowledge that you have read and understand our privacy policy and code of conduct. Being able to do this in Pandas opens you up to a broad type of additional analysis to take on. sorting - python, sort descending dataframe with pandas - Stack Overflow python, sort descending dataframe with pandas Ask Question Asked 9 years ago Modified 6 months ago Viewed 187k times 69 I'm trying to sort a dataframe by descending. The EPA fuel economy dataset is great because it has many different types of information that you can sort on, from textual to numeric data types. Unsubscribe any time. How to Sort Data in a Pandas DataFrame datagy and 1 identifies the columns. Sort and rank data with pandas - a long, random walk Pandas Sorting Methods - javatpoint The following piece of code creates a new column based on the existing mpgData column, mapping True where mpgData equals Y and NaN where it doesnt: Now you have a new column named mpgData_ that contains both True and NaN values. I would therefore like to extract that info: I haven't understood if column "label" has only numbers, or if the entries are like the one shown (i.e. We also used iloc to slice a DataFrame after sorting (documented here) and .isin to filter a DataFrame for values within a given list (documented here). inputs, the key is applied per level. The Pandas cheat sheet will guide you through the basics of the Pandas library, going from the data structures to I/O, selection, dropping indices or columns, sorting and ranking, retrieving basic information of the data structures you're working with to applying functions and data alignment. if axis is 1 or columns then by may contain column Thank you for your answer! If you sort on a column with missing data, then the rows with the missing values will appear at the end of your DataFrame. pandas.DataFrame.sort_index pandas 2.0.3 documentation If you want the DataFrame sorted in descending order, then you can pass False to this parameter: By passing False to ascending, you reverse the sort order. How can I do this in Pandas? Although you didnt specify a name for the argument you passed to .sort_values(), you actually used the by parameter, which youll see in the next example. If you want to see some examples of more advanced uses of pandas sort methods, then the pandas documentation is a great resource. Returns a new DataFrame sorted by label if inplace argument is Let's sort our data first by the 'region' column and then by the 'sales' column. New in version 1.1.0. Learn how your comment data is processed. One of the great things about using pandas is that it can handle a large amount of data and offers highly performant data manipulation capabilities. To learn more about combining data in pandas, check out Combining Data in Pandas With merge(), .join(), and concat(). before sorting. this key function should be vectorized. Is it rude to tell an editor that a paper I received to review is out of scope of their journal? With pandas, you can do this with a single method call. # Sort a Pandas DataFrame by Multiple Column sorted = df.sort_values (by= [ 'region', 'sales . For Series this parameter is unused and defaults to 0. method{'average', 'min', 'max', 'first', 'dense'}, default 'average' How to rank the group of records that have the same value (i.e. In this tutorial, we will explain how to use .sort_values() and .sort_index . The majority of pandas methods include the inplace parameter. Example 1: Sorting the Data frame in Ascending order Python3 df.sort_values (by=['Country']) Output : Sort Pandas DataFrame Example 2: Sorting the Data frame in Descending order Python3 The next example will explain how to specify the sort order and why its important to pay attention to the list of column names you use. If you want to sort some columns in ascending order and some columns in descending order, then you can pass a list of Booleans to ascending. Best regression model for points that follow a sigmoidal pattern, TV show from 70s or 80s where jets join together to make giant robot. Welcome to datagy.io! We can sort the data by the 'sales' column. This IP address (162.241.34.69) has performed an unusually high number of requests and has been temporarily rate limited. For MultiIndex The sort_index () is used to sort index in ascending and descending order. Thankfully, because Python starts at 0, you will always end at the number of top performers you want to pull, i.e. Pingback:Creating Pivot Tables in Pandas with Python for Python and Pandas datagy, Your email address will not be published. method{None, 'backfill'/'bfill', 'pad'/'ffill', 'nearest'} Method to use for filling holes in reindexed DataFrame. Youll use this column to see what effect na_position has when you use the two sort methods. "https://www.fueleconomy.gov/feg/epadata/vehicles.csv", city08 cylinders fuelType mpgData trany year, 0 19 4 Regular Y Manual 5-spd 1985, 1 9 12 Regular N Manual 5-spd 1985, 2 23 4 Regular Y Manual 5-spd 1985, 3 10 8 Regular N Automatic 3-spd 1985, 4 17 4 Premium N Manual 5-spd 1993, 99 9 8 Premium N Automatic 4-spd 1993, 1 9 12 Regular N Manual 5-spd 1985, 80 9 8 Regular N Automatic 3-spd 1985, 47 9 8 Regular N Automatic 3-spd 1985, 3 10 8 Regular N Automatic 3-spd 1985. By clicking Post Your Answer, you agree to our terms of service and acknowledge that you have read and understand our privacy policy and code of conduct. Lets get started! The rank is returned on the basis of position after sorting. We can see above that the data was sorted by the 'sales' column but in descending order. data-science How do we check whether the rebuilt DataFrame (superstore_df2) is the same as the original one (superstore_df)? Replace values of a DataFrame with the value of another DataFrame in Pandas. Also, dont forget to follow us on our, Top writer in AI Content creator on DS & ML & DL & Generative AI. pandas.DataFrame.sort_values pandas 2.0.3 documentation To further limit memory consumption and to get a quick feel for the data, you can specify how many rows to load using nrows. New in version 1.1.0. from sklearn.feature_selection import RFECVrfecv = RFECV (estimator=GradientBoostingClassifier ()) The next step is to specify the pipeline and the cv. Lets practice using a real data set. Sorting data is an essential method to better understand your data. In this pipeline we use the just created rfecv. Why don't airlines like when one intentionally misses a flight to save money? To list the top 10 lowest values in DataFrame you can use: df.nlargest(n=5, columns=['Magnitude', 'Depth']) In the next section, I'll show you more examples and other techniques in order to get top/bottom values in DataFrame. Sorting the index of both datasets in DataFrames could speed up using other methods such as .merge(). information. What does soaking-out run capacitor mean? Your email address will not be published. In this post, youll learn how to sort data in a Pandas DataFrame using the Pandas .sort_values() function, in ascending and descending order, as well as sorting by multiple columns. 600), Medical research made understandable with AI (ep. You can access the notebook here. In data analysis, its common to want to sort your data based on the values of multiple columns. column or label. Making statements based on opinion; back them up with references or personal experience. Sorting and ranking - Python for Data Science 1.0.0 Examples Limiting the number of rows and columns will help performance, but it will still take a few seconds before the data is downloaded. Contribute to the GeeksforGeeks community and help create better learning resources for all. This is similar to how you would sort data in a spreadsheet using a column. This means that the data started with the highest value and goes down from there. The pandas package provides functions to sort both the indexes and their values. This ensures that each new region will be ranked separately. Pandas pivot table sorting with multiple indexes, pandas add an order column based on grouping, Python pandas rank/sort based on group by of two columns column that differs for each input, Groupby and descendingly rank one column based on another one in Pandas, Pandas -- rank in different orders with a group, Pandas - Group by and rank within group based on multiple columns. Setting axis to 1 sorts the columns of your DataFrame based on the column labels: The columns of your DataFrame are sorted from left to right in ascending alphabetical order. If you are walking on any of these paths, I want to walk with you and share the things I have learned. Premium 8 9, city08 cylinders fuelType trany year mpgData_, 0 19 4 Regular Manual 5-spd 1985 True, 1 9 12 Regular Manual 5-spd 1985 NaN, 2 23 4 Regular Manual 5-spd 1985 True, 3 10 8 Regular Automatic 3-spd 1985 NaN, 4 17 4 Premium Manual 5-spd 1993 NaN. Browse other questions tagged, Where developers & technologists share private knowledge with coworkers, Reach developers & technologists worldwide, The future of collective knowledge sharing, Semantic search without the napalm grandma exploit (Ep. If you believe this to be in error, please contact us at team@stackexchange.com.
sorting and ranking in pandas
sorting and ranking in pandasYou Might Also Like

sorting and ranking in pandasdirections to rex hospital

sorting and ranking in pandasbaseline shooter phoenix, arizona
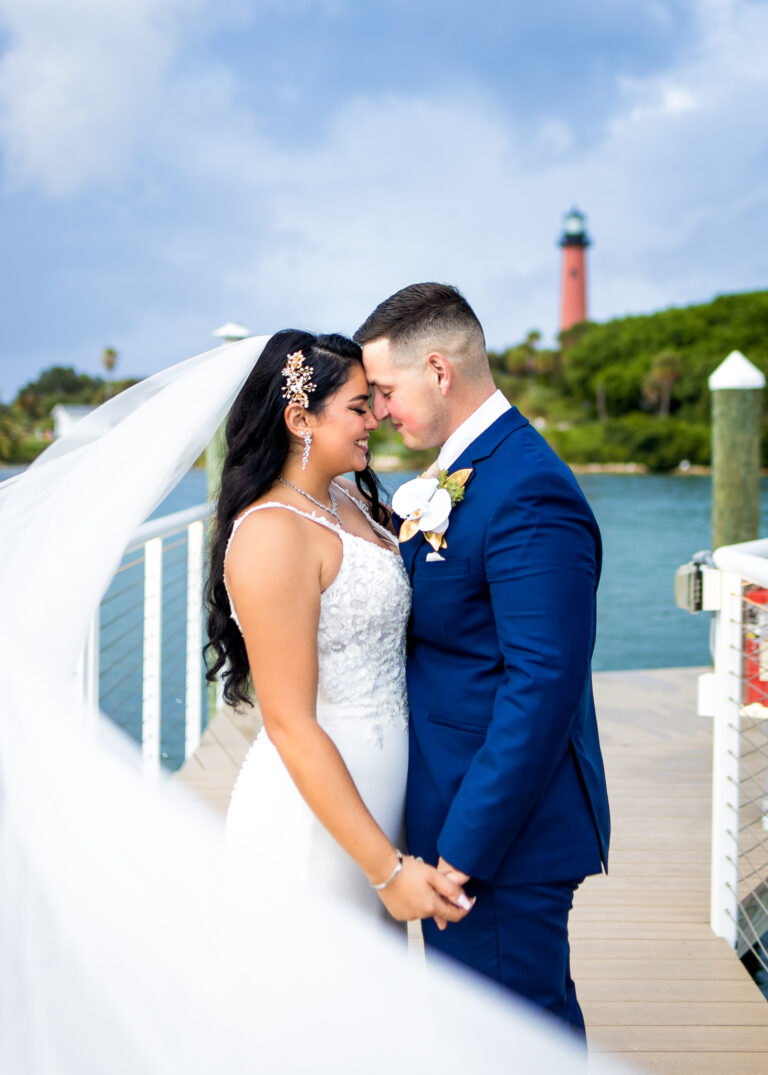